Remember, network boundaries are created based on imports, not based on ancestors. This means that any component type can be the parent or child of any other component type.
I am a server component
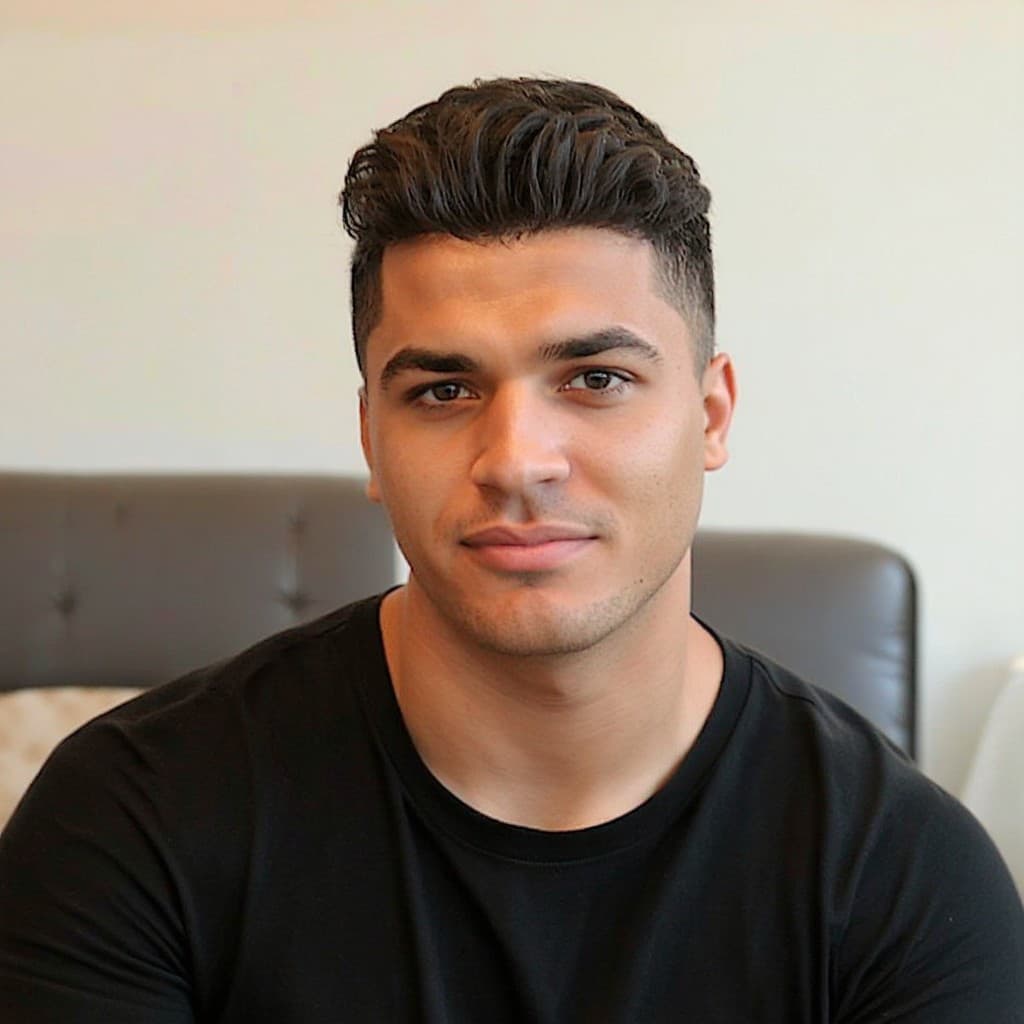
Dr. Tommie Stoltenberg PhD
Direct Mobility Supervisor
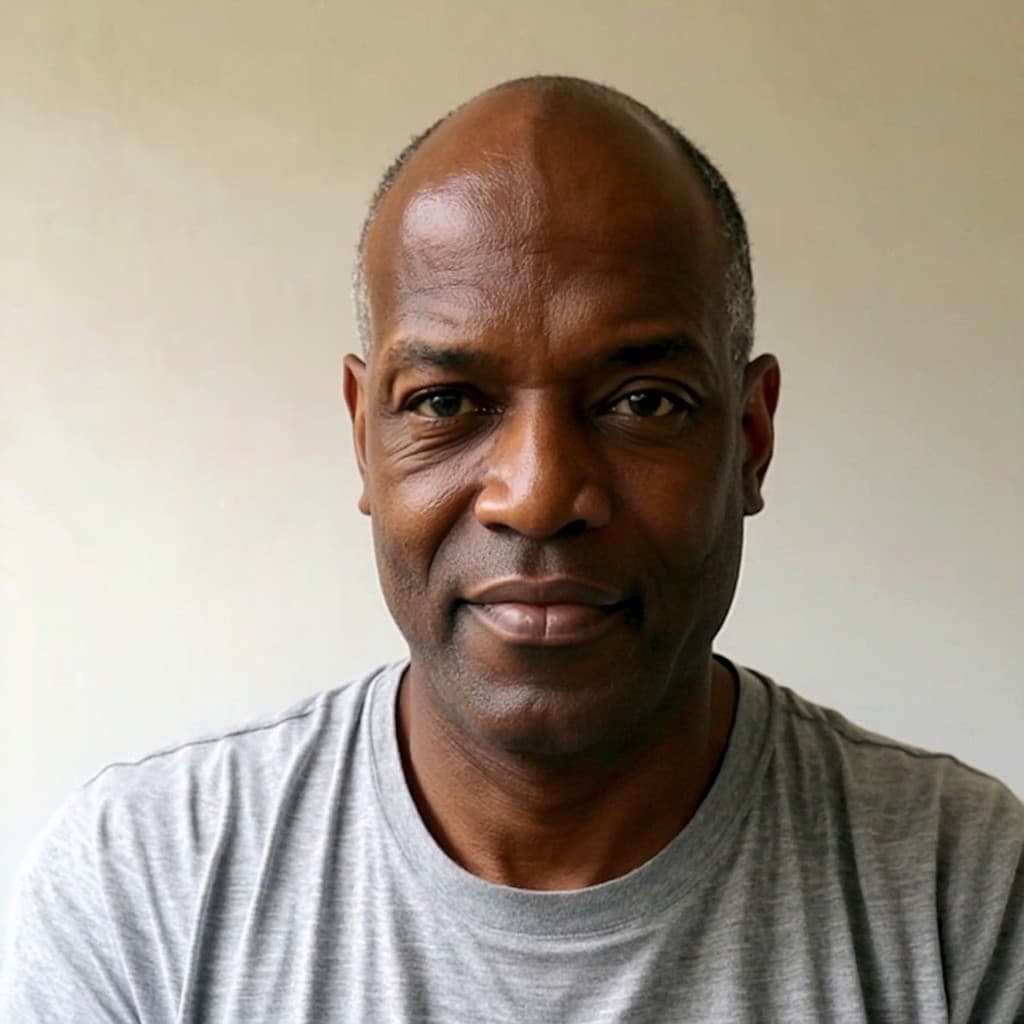
Lester Labadie
Principal Data Engineer
Hi, shared component here. Take note of my environment.
We can pass Server Components "through" child Client Components without creating a network boundary:
I can do client things: 0
Hi, shared component here. Take note of my environment.
Hi, shared component here. Take note of my environment.
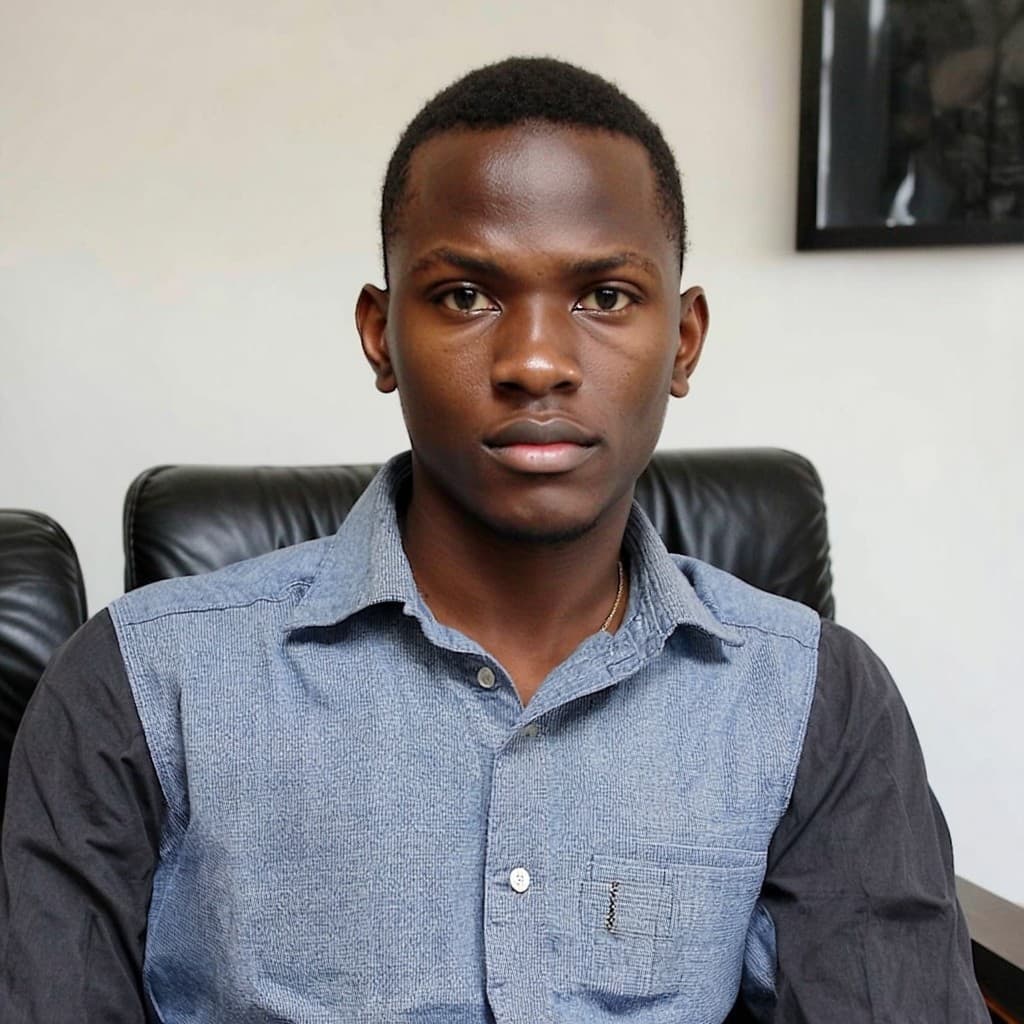
Jesse Kshlerin
Forward Creative Strategist
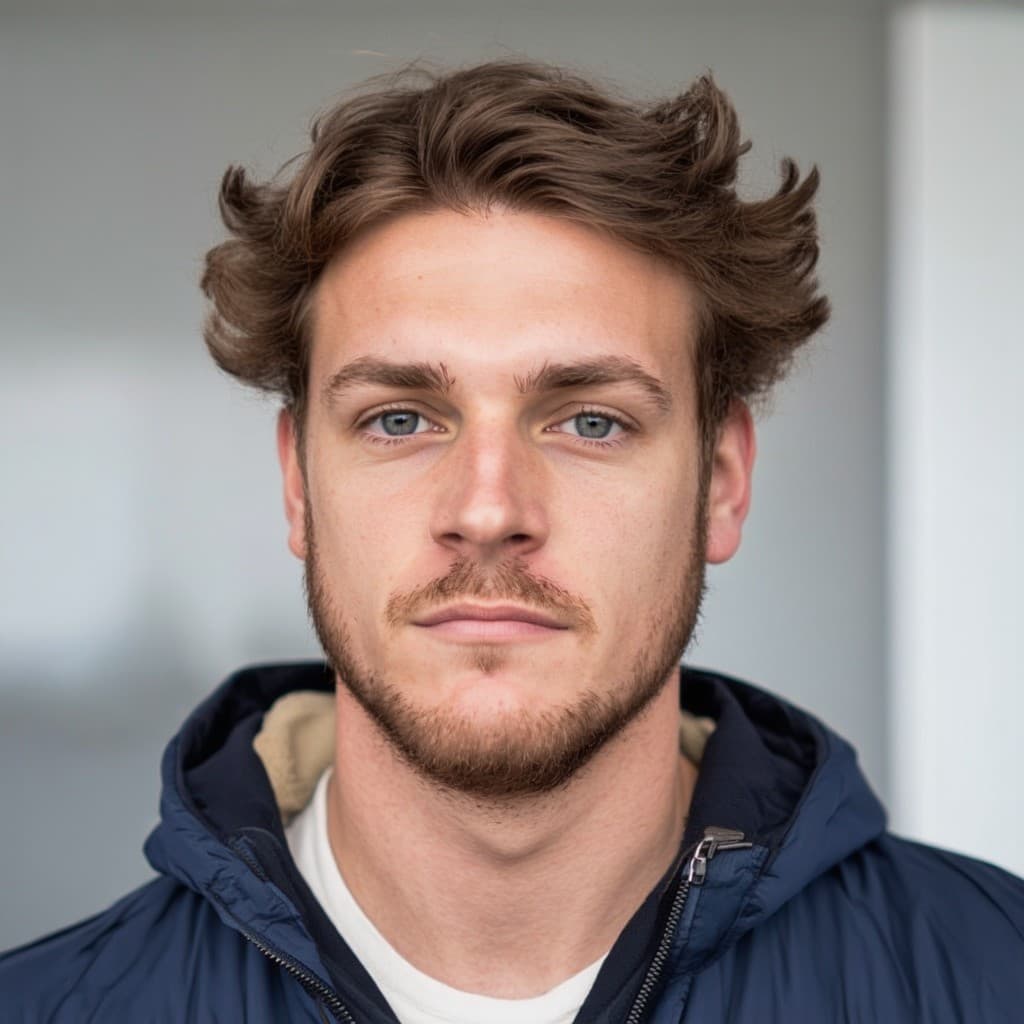
Christian Murray
Direct Directives Assistant
A more complex example, passing a Server Component as a prop and deeply nesting:
I can do client things: 0
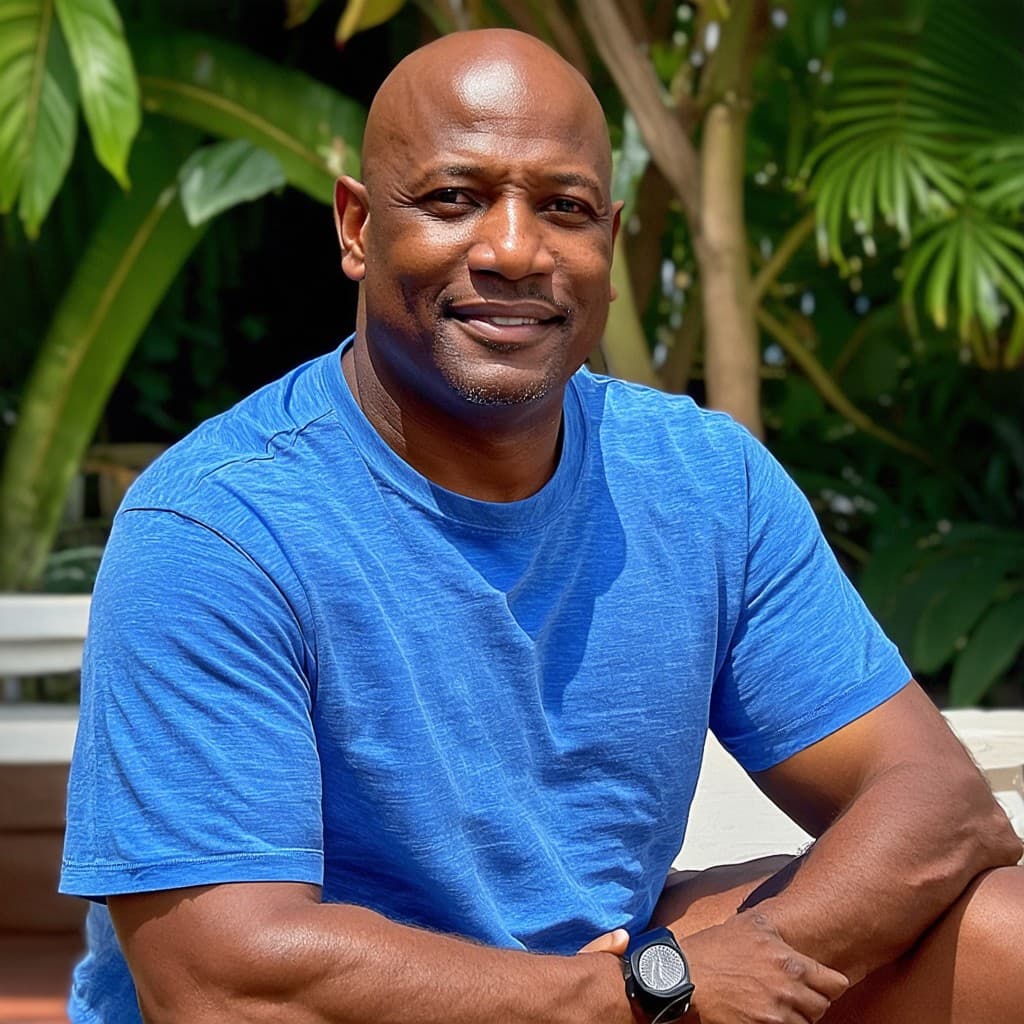
Dave Kovacek
National Assurance Agent
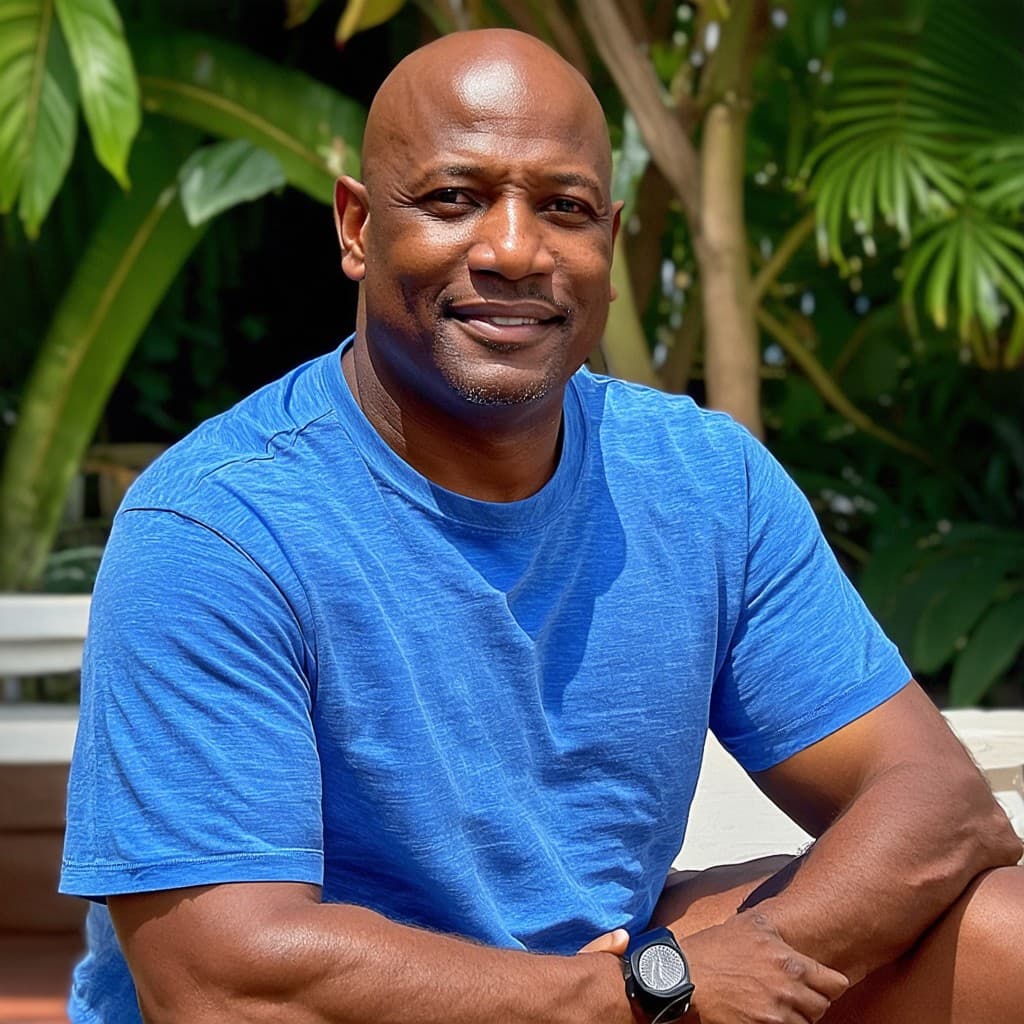
Samuel Hills
National Interactions Technician
Hi, shared component here. Take note of my environment.
Hi, shared component here. Take note of my environment.
I can do client things: 0
Hi, shared component here. Take note of my environment.
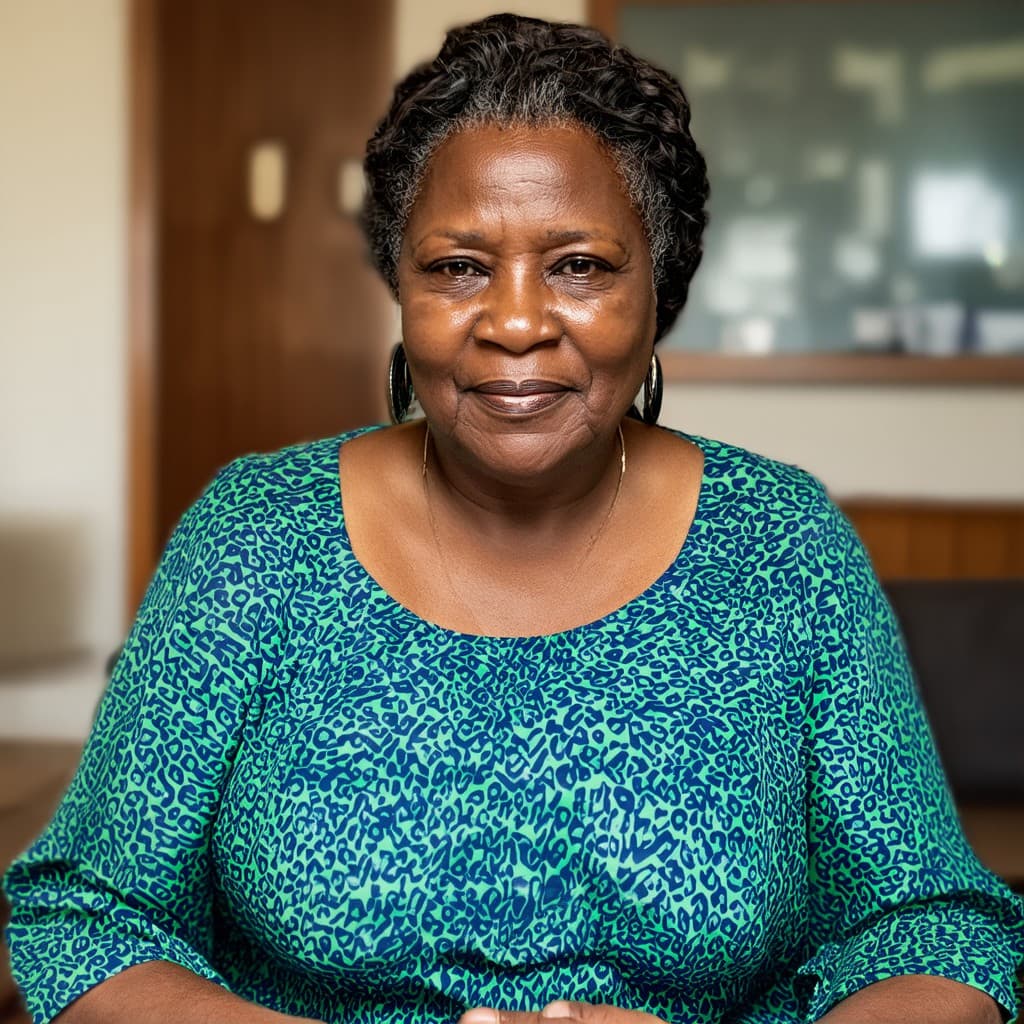
Katrina Yost III
Direct Directives Associate
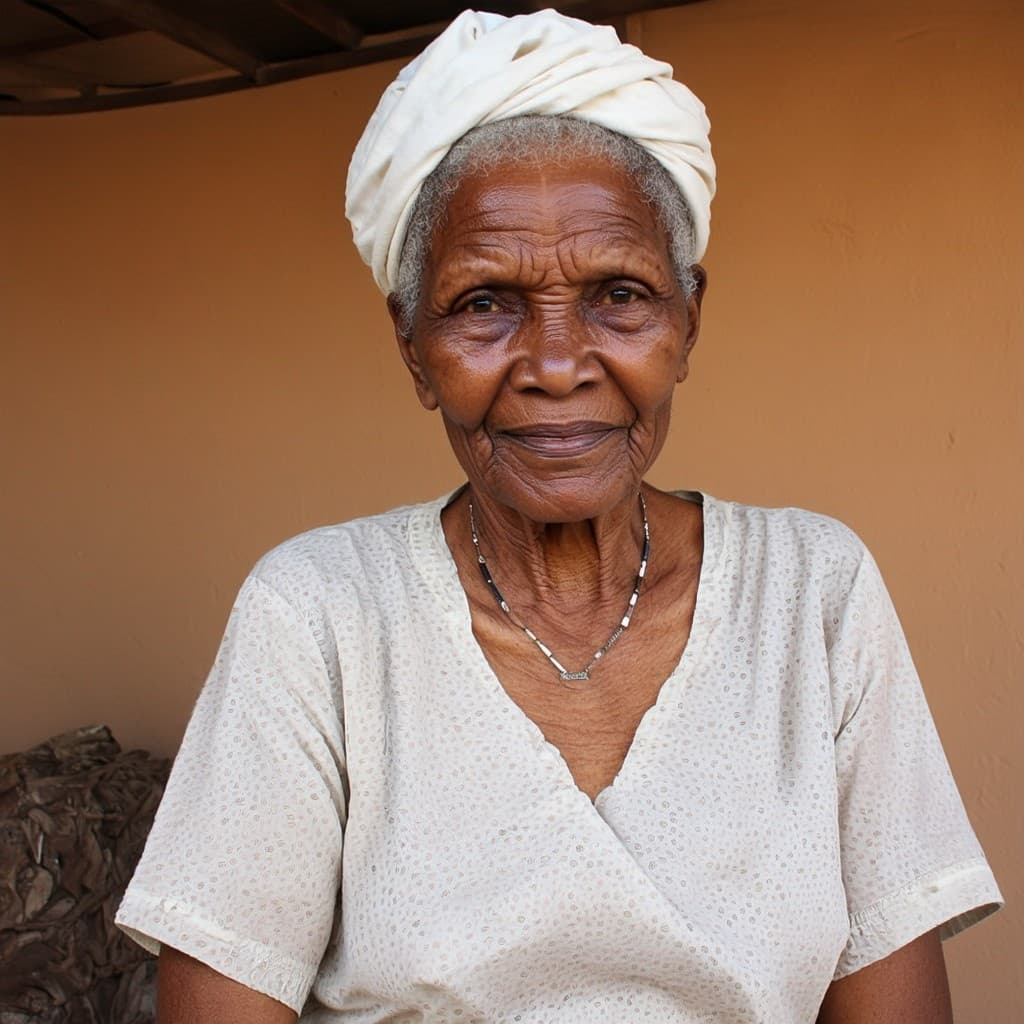
Dr. Joan Raynor
Human Program Designer