React Server Components can use the cache
function to memoize expensive computations or data fetching operations. This is similar to useMemo
on the client side, but it works on the server and persists between components that use the same cached function.
The cache
function returns a cached result instead of re-executing the computation. This mechanism is particularly useful for:
- Server Components that appear many times in the tree
- Expensive database queries
- Complex calculations
- API calls that return the same data for the same parameters
Note that the cache persists when rendering components during the same server request, but it is invalidated upon a new server request.
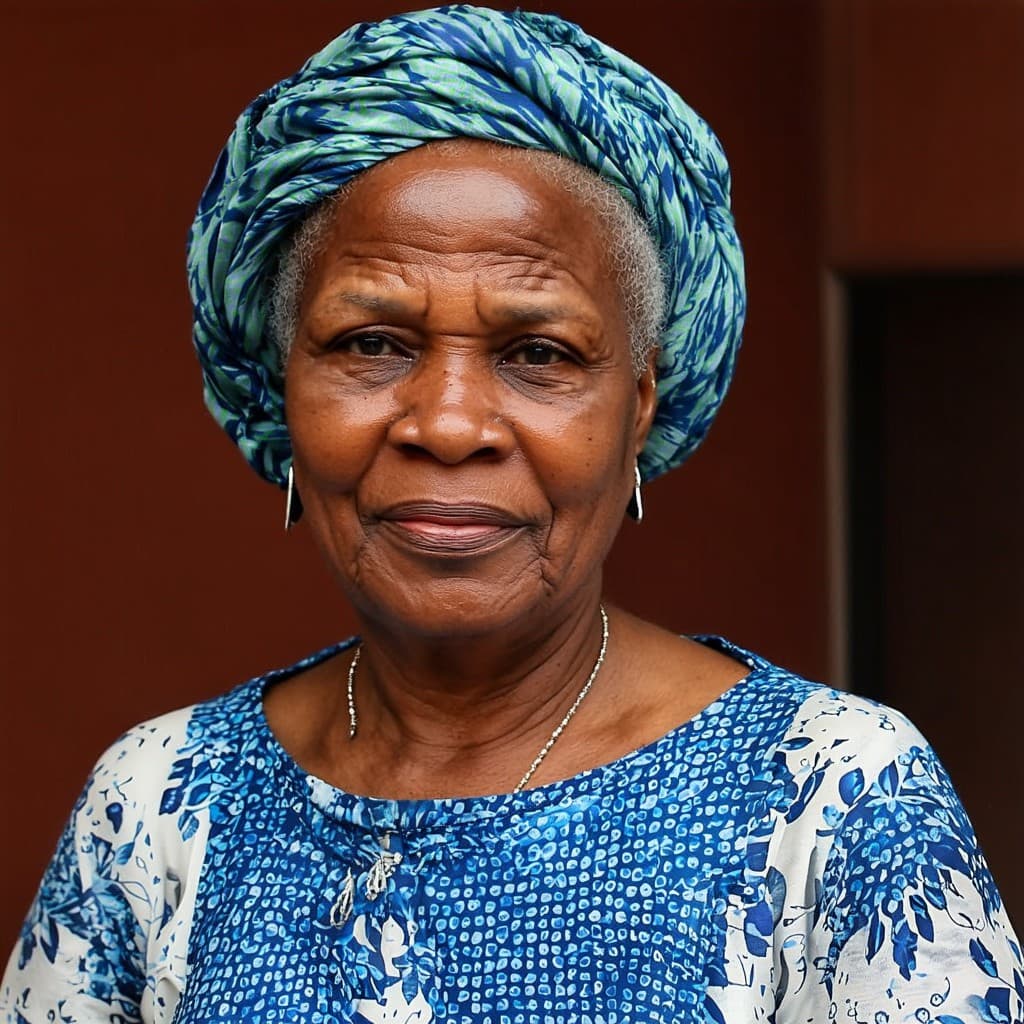
Ollie Gusikowski
Investor Quality Director
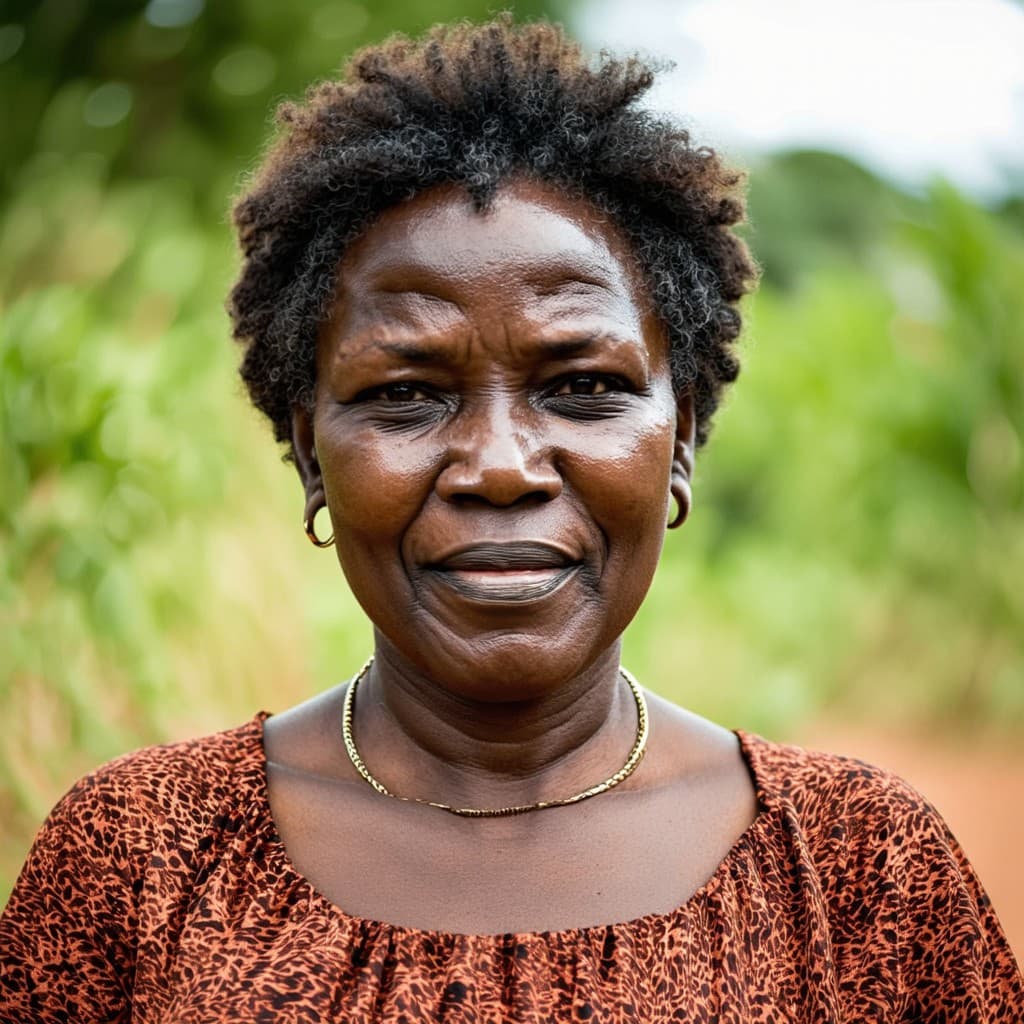
Charlotte Champlin
Central Infrastructure Assistant
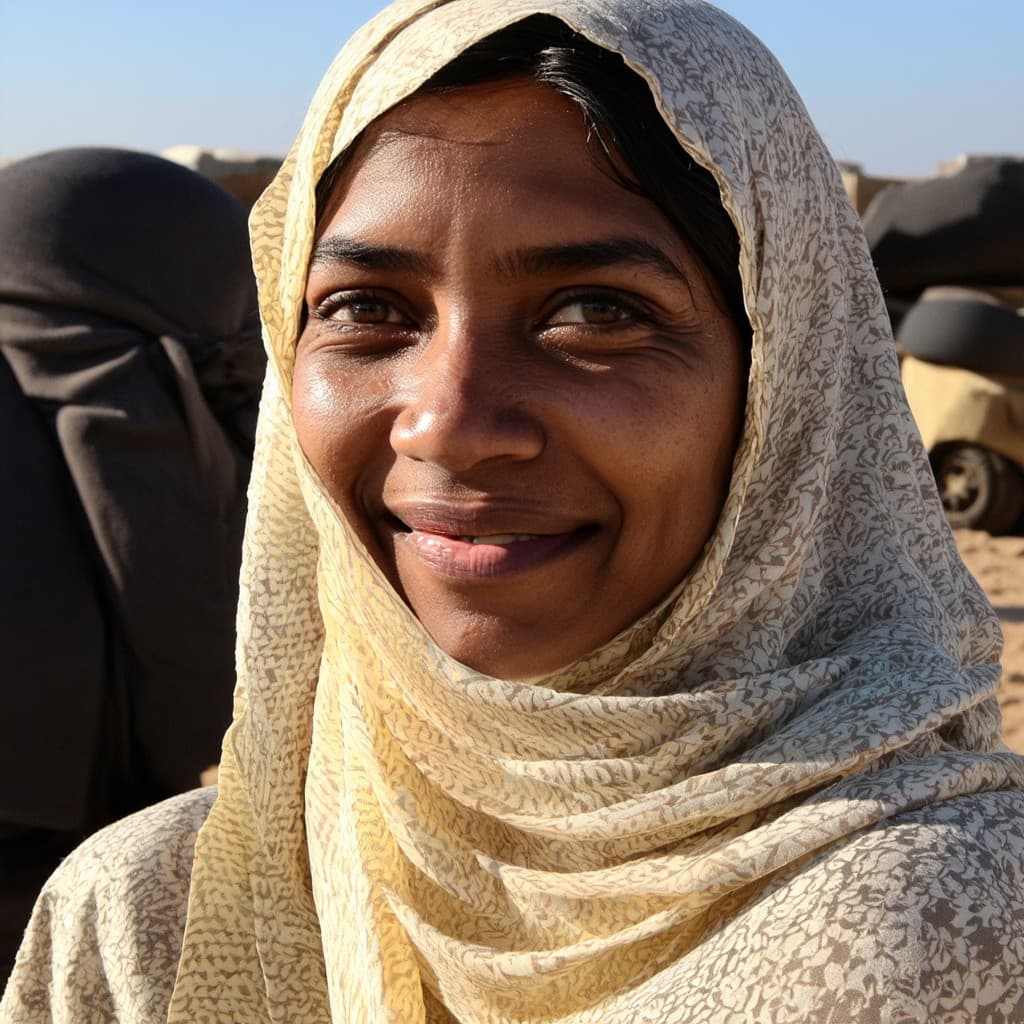
Robin Corkery
Customer Research Developer
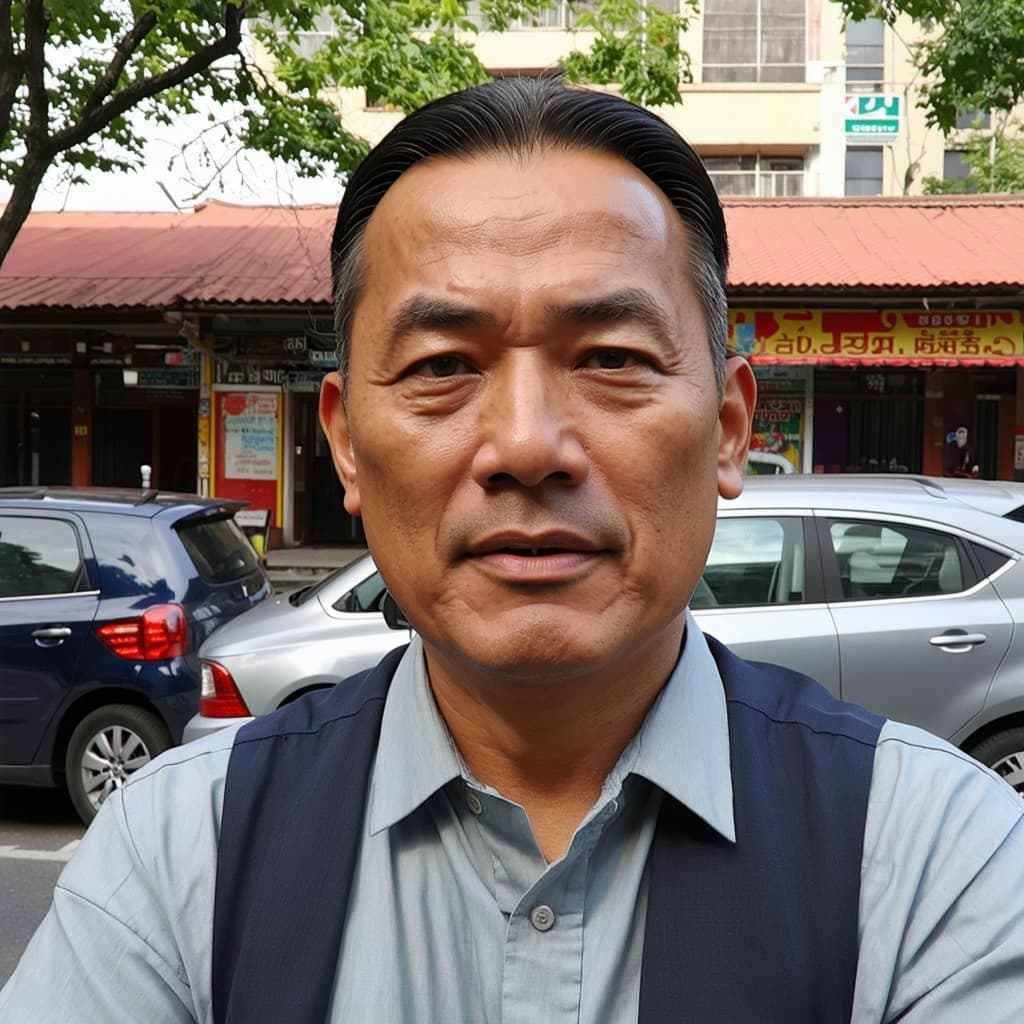
Tracy Cremin
Product Marketing Strategist
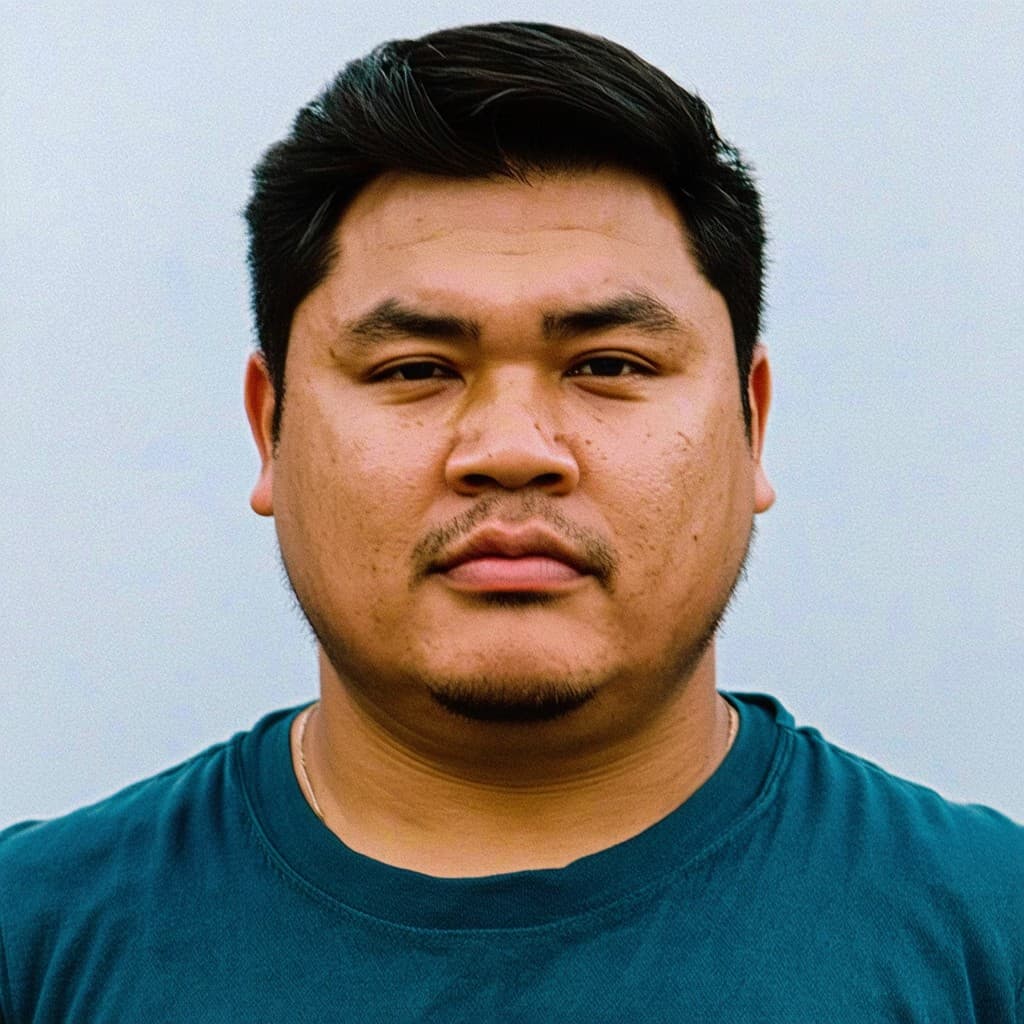
Kyle Farrell V
Dynamic Interactions Producer
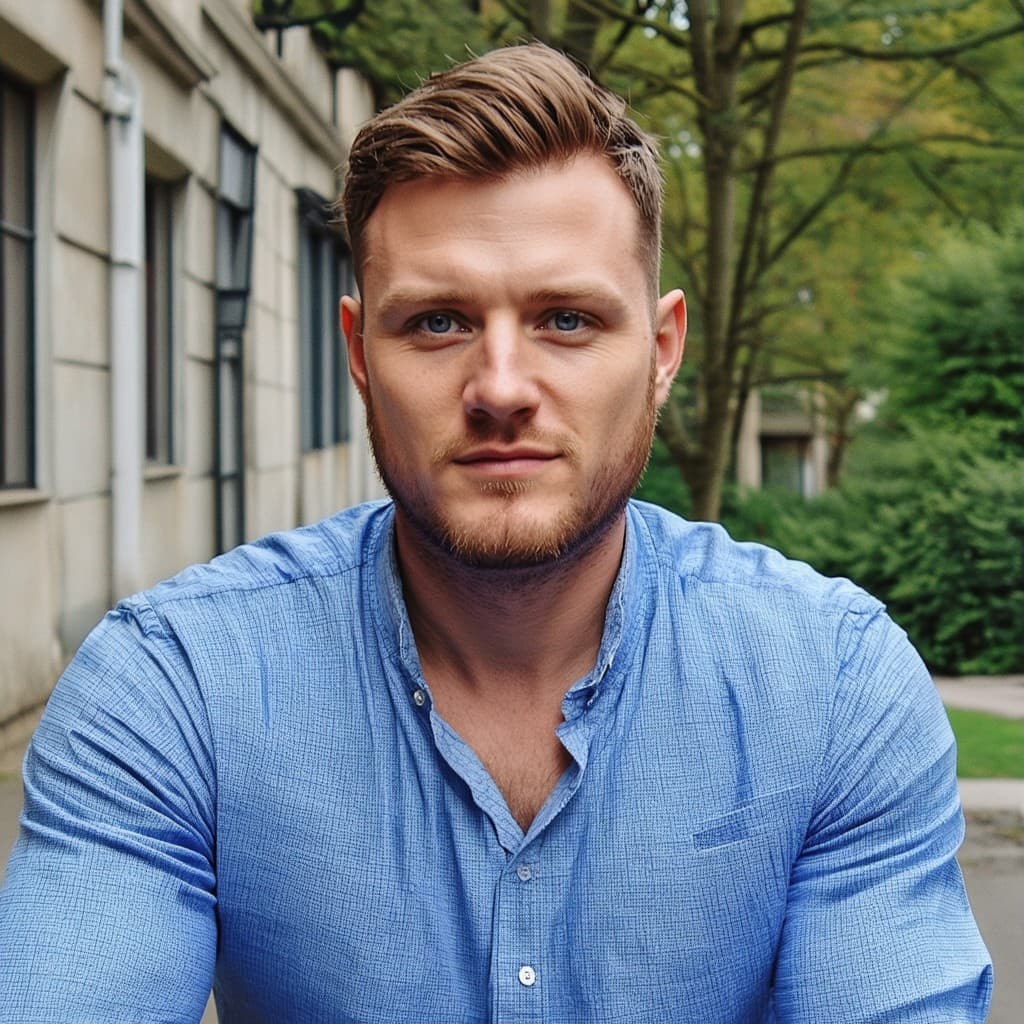
Sherman Mitchell
Dynamic Web Liaison
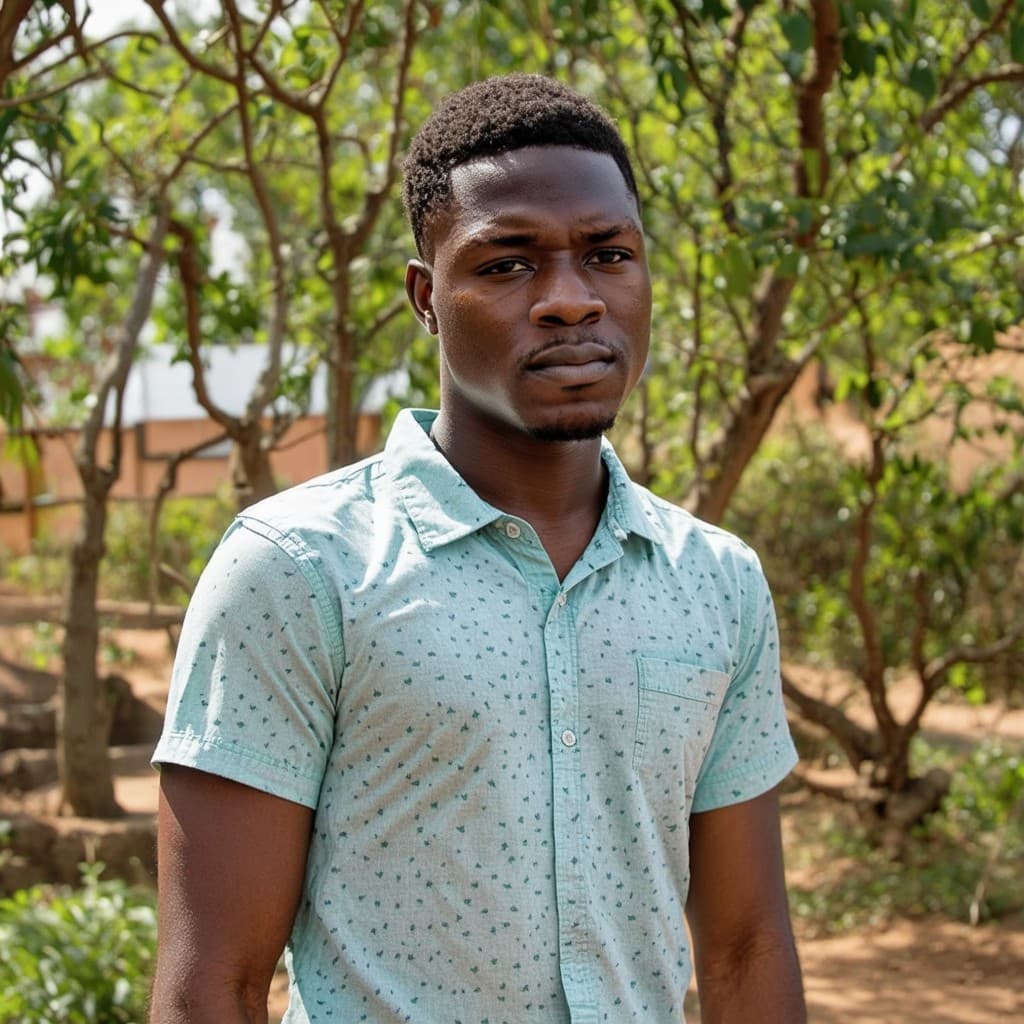
Brent Stracke
National Quality Specialist
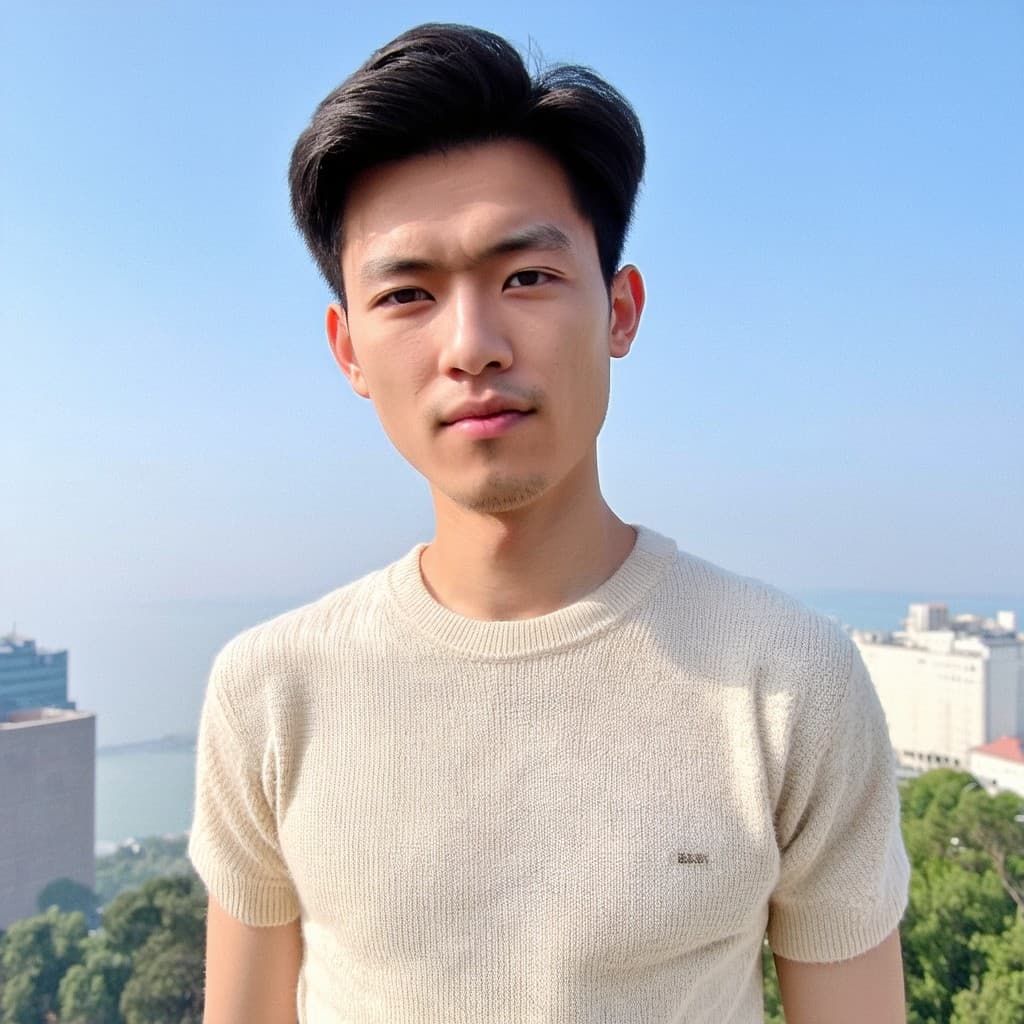
Dr. Marvin Padberg
Principal Assurance Specialist
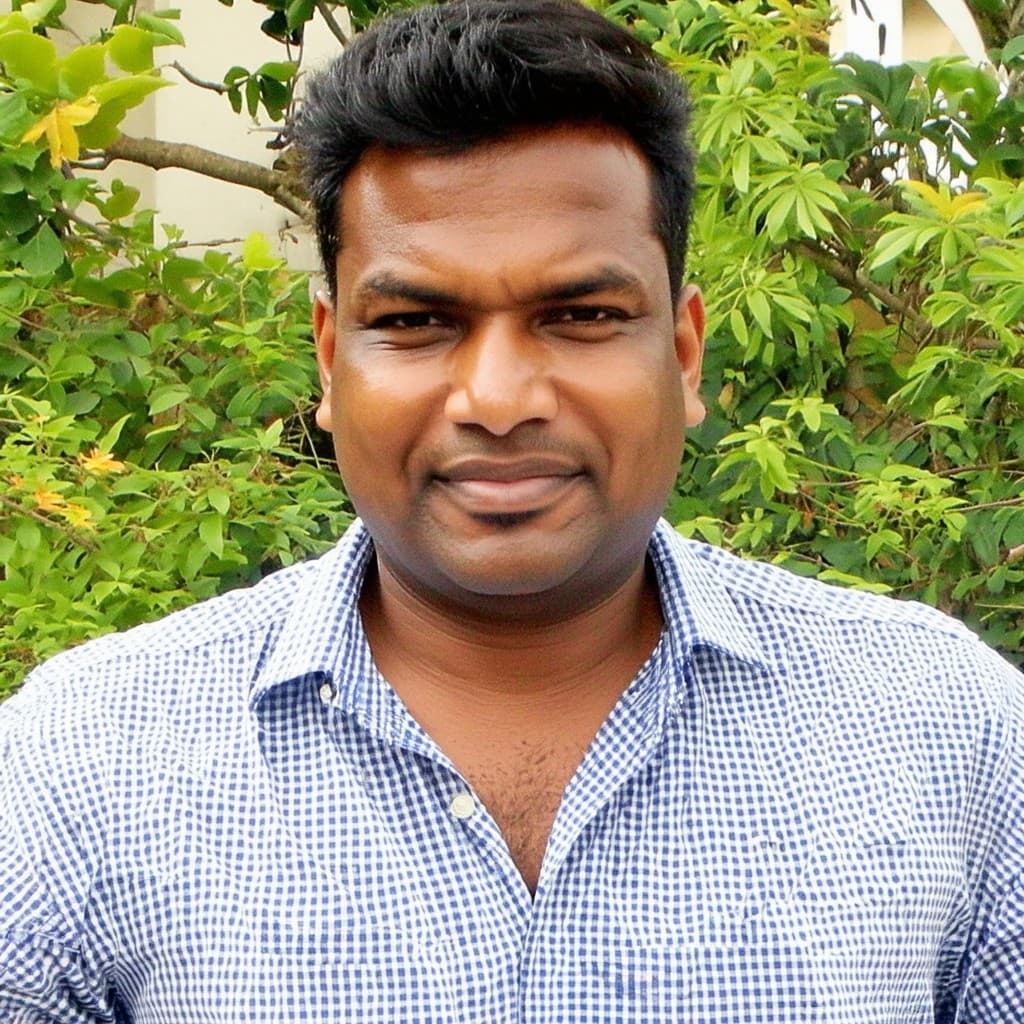
Dr. Jeff Kautzer
Direct Mobility Coordinator
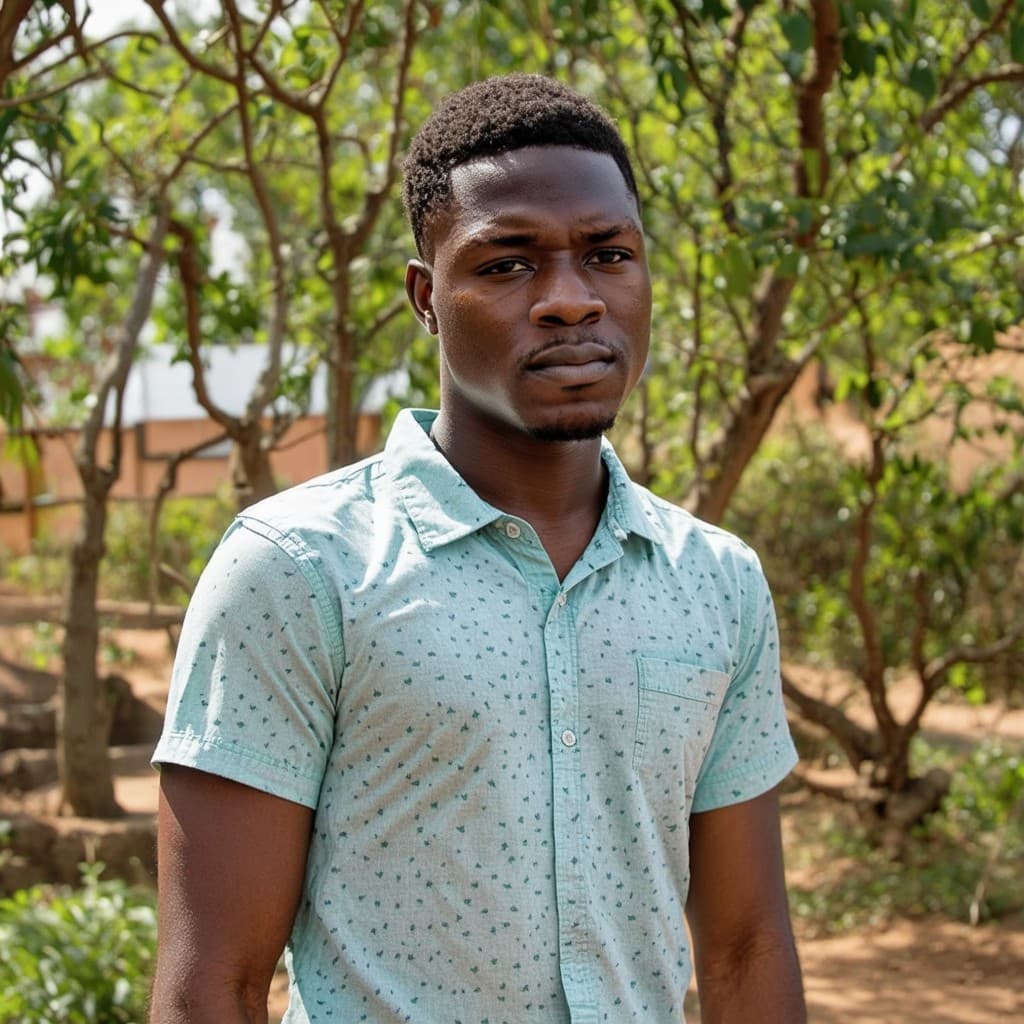
Brent Stracke
National Quality Specialist
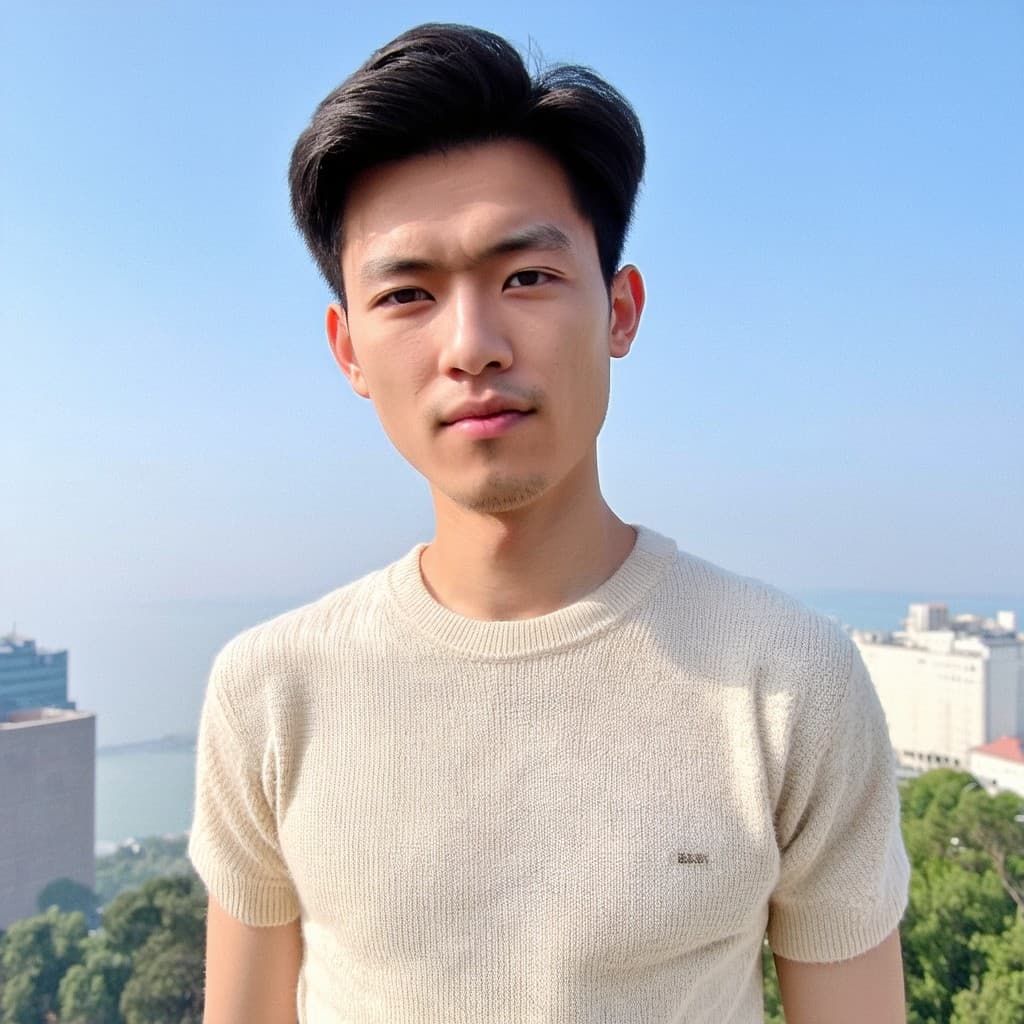
Dr. Marvin Padberg
Principal Assurance Specialist
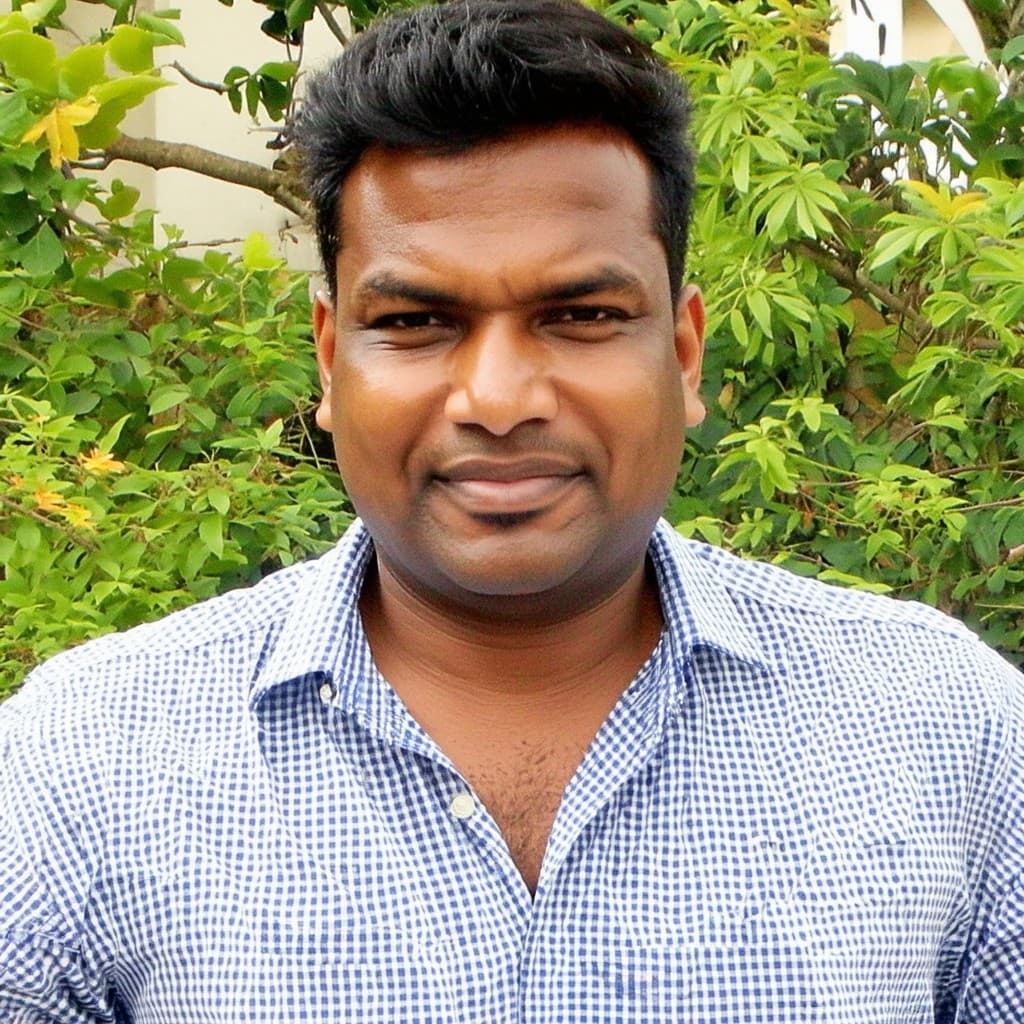
Dr. Jeff Kautzer
Direct Mobility Coordinator